2
This program prompts the user to input a phrase, then counts the number of vowels and consonants present in that phrase, ignoring uppercase letters and considering only alphabetical characters.
```py
def count_vowels_consonants(phrase):
vowels = 0
consonants = 0
# Converting the phrase to lowercase for easier comparison
phrase = phrase.lower()
# Defining a list of vowels
vowels_list = ['a', 'e', 'i', 'o', 'u']
# Iterating over each character in the phrase
for character in phrase:
# Checking if the character is a letter
if character.isalpha():
# Checking if the character is a vowel
if character in vowels_list:
vowels += 1
else:
consonants += 1
return vowels, consonants
# Asking the user to input a phrase
user_phrase = input("Enter a phrase: ")
# Calling the function and storing the result
num_vowels, num_consonants = count_vowels_consonants(user_phrase)
# Displaying the results
print("Number of vowels:", num_vowels)
print("Number of consonants:", num_consonants)
```
I leave you with another challenge, which you can answer in the comments.
**Challenge**
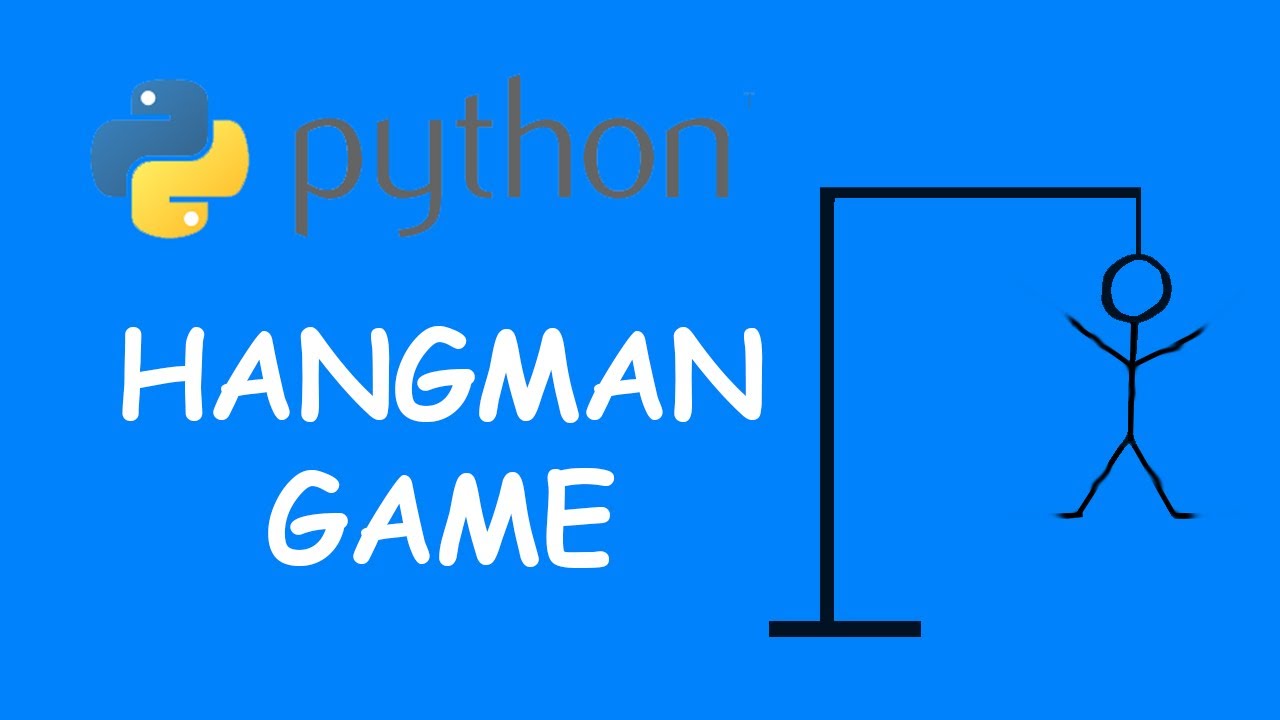
* Hangman: Implement the classic game of hangman, where the player must guess a hidden word before exhausting all attempts.👇
id just go ahead and presume this is malware since theres no description at all