
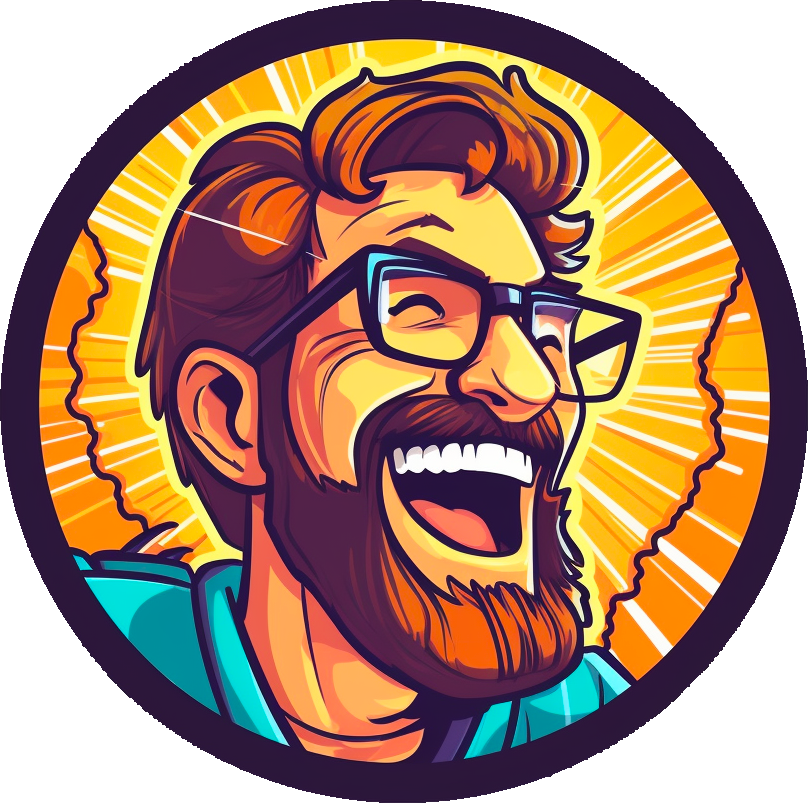
I think the problem is that many introductory examples use unwrap
, so many beginner programmers don’t get exposed to alternatives like unwrap_or
and the likes.
I think the problem is that many introductory examples use unwrap
, so many beginner programmers don’t get exposed to alternatives like unwrap_or
and the likes.
I’m a OOP programmer.
I wrap everything within Arc<Mutex<>>
.
I’m a happy dev.
This is why I rarely use AI for coding. How to put my thoughts into code is not my main concern. My main concern is that another person should understand my thoughts when reading the code.
HL2 still holds up after 10+ playthroughs.
Last time I played it was when the commentary track was added. Played 80% of the game in one sitting because I was so hooked.
That’s how these ”self driving vehicle” companies generally works.
Job description: we’re looking for someone with experience in deploying cutting edge machine learning systems, preferably PhD.
Actual job: Excel spreadsheet
And after all that it is discovered that it was the wrong solution all along because the requirements were poorly specified, so the process must be started all over again
Product Manager: Make a step by step guide of how they think the lightbulb is going to be fixed without explicitly mentioning the broken lightbulb.
jQuery got popular because Internet Explorer, Firefox, Chrome and other browsers weren’t exactly cross compatible. Writing vanilla JS was risky business in that sense.
It also supported AJAX across all major browsers, which meant the website could make API requests without reloading the entire page. It was super revolutionary to press a button and it only changed a part of the page.
Then Angular and React took it a step forward and that’s where we are now.
JavaScript frameworks are invented because pure HTML and CSS suck for dynamically loaded pages, and vanilla JavaScript suck in general.
What’s not shown is that the car doesn’t have an engine. Management was really eager to release it to the customer. Don’t worry, it’s planned to get fixed later (spoiler: it’s never going to get fixed).
Rust and Cargo were built to be in a symbiosis with each other.
NPM is an afterthought of a rushed language.
I believe it’s mostly cultural. Alcoholic beverages are as old as civilization itself. We drink alcohol because that has been the social norm for thousands of years.
But there’s probably something about it being a beverage as well. It goes along well with food because of this, which makes it a tasteful alternative to water.
Then you haven’t seen bad documentation (or had that sex you regret).
There’s also ”we do machine learning”, which usually translates to ”someone trained an SVM model 10 years ago”.
It’s artificial in the sense that it’s not real. It’s “not real” intelligence imitating as “real” intelligence.
The derivative of an exponential is exponential. The relative difference between -1 and -2 is the same as 1 and 2.
I’d say the development is exponential. Compare what we had 4 years ago, 2 years ago and now. 4 years ago it was inconceivable that an AI model could generate any convincing video at all. 2 years ago we laughed at Will Smith eating pasta. Today we have Veo 3 which generates videos with sound that are near indistinguishable from real life.
It’s not going to be long until you regularly see AI generated videos without realizing it’s AI.
Arnold jumps into the pool of lava to destroy all hardware that could potentially be used to create Skynet. The inventor of Skynet is also killed with all the source code.
It’s heavily implied they managed to prevent Skynet in that timeline. But yeah, money.
Static lifetimes confused me when I started learning rust. The error message guides the developer to the wrong direction.
It took me a while to realize that just using
Arc
is sufficient in most of those cases.